|
Post by bigbass on Feb 2, 2014 21:07:42 GMT 1
Hey John sdlkeys.cI tried this and it works I could port it for BaCon use too Joe
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 2, 2014 21:14:24 GMT 1
Thanks Joe.
SDL gets cranky if you leave the engine running without checking in.
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 2, 2014 22:35:48 GMT 1
I think we are going to need a BBC compatible RND function as the BaCon (as well as SB) didn't fit the bill. Here is the fern.bac example that goes into an endless loop. (had to logout to exit the process / unkillable)
' Fern
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS m SDL bbc PROTO init_screen, emulate_mode, emulate_origin, emulate_off, emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen, SDL_WM_SetCaption
DECLARE a,b,c,d,e,f,x,y,r,newx, newy TYPE FLOATING
init_screen() SDL_WM_SetCaption("BaCon BBC Fern", 0) emulate_mode(31) emulate_origin(200, 100) emulate_off() emulate_gcol(0, 10) x=0 y=0 FOR i = 1 TO 80000 SEED NOW r = RANDOM(1) IF r <= 0.1 THEN a = 0 b = 0 c = 0 d = 0.16 e = 0 f = 0 ENDIF IF r > 0.1 AND r <= 0.86 THEN a = .85 b = .04 c = -.04 d = .85 e = 0 f = 1.6 ENDIF IF r > 0.86 AND r <= 0.93 THEN a = .2 b = -.26 c = .23 d = .22 e = 0 f = 1.6 ENDIF IF r> 0.93 THEN a = -.15 b = .28 c = .26 d = .24 e = 0 f = .44 ENDIF newx = a * x + b * y + e newy = c * x + d * y + f x = newx y = newy emulate_move(600 + 96 * x, 32 + 96 * y) emulate_draw(600 + 96 * x, 32 + 96 * y) NEXT i INPUT wait$ end_screen()
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 2, 2014 22:41:50 GMT 1
Here is my BBC RND solution. (hacked Brandy code)
// BBC_RND besFUNCTION(BBC_RND) DIM AS int32 value; besARGUMENTS("i") AT value besARGEND IF (value < 0) THEN lastrandom = value; srand((unsigned)time(NULL)); value=rand(); randomoverflow = 0; besRETURN_LONG(value); ELSE_IF (value EQ 0) THEN besRETURN_DOUBLE(randomfraction()); ELSE_IF (value EQ 1) THEN nextrandom(); besRETURN_DOUBLE(randomfraction()); ELSE nextrandom(); besRETURN_LONG(TOINT((randomfraction()*TOFLOAT(value))+1)); END_IF besEND
#define TOFLOAT(x) ((float64)(x)) #define TOINT(x) ((int32)(x))
DIM AS static int32 lastrandom; DIM AS static int32 randomoverflow; DIM AS static float64 floatvalue;
FUNCTION LOCAL float64 randomfraction(void) BEGIN_FUNCTION uint32 reversed = ((lastrandom>>24)&0xFF)|((lastrandom>>8)&0xFF00)|((lastrandom<<8)&0xFF0000)|((lastrandom<<24)&0xFF000000); RETURN_FUNCTION(TOFLOAT(reversed) / 4294967296.0); END_FUNCTION
LOCAL SUB nextrandom(void) BEGIN_SUB DIM AS int n; FOR (n = 0 TO n < 32 STEP INCR n) BEGIN_FOR int newbit = ((lastrandom>>19) ^ randomoverflow) & 1; randomoverflow = lastrandom>>31; lastrandom = (lastrandom<<1) | newbit; NEXT END_SUB
|
|
|
Post by vovchik on Feb 2, 2014 22:49:31 GMT 1
Dear John
RANDOM() will never generate a float. You ahve to use something like this
DEF FN QRND = (double)(RANDOM(1000) / 1000.0)
which will behave like QBASIC etc.
With kind regards, vovchik
PS. And, now it seems to work fine:
' Fern
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS m SDL bbc PROTO init_screen, emulate_mode, emulate_origin, emulate_off, emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen, SDL_WM_SetCaption DECLARE i TYPE long DECLARE a,b,c,d,e,f,x,y,r,newx, newy TYPE float SEED NOW DEF FN QRND = (float)(RANDOM(1000) / 1000.0)
init_screen() SDL_WM_SetCaption("BaCon BBC Fern", 0) emulate_mode(31) emulate_origin(200, 100) emulate_off() emulate_gcol(0, 10) x = 0 y = 0 FOR i = 1 TO 80000 r = QRND IF r <= 0.1 THEN a = 0 b = 0 c = 0 d = 0.16 e = 0 f = 0 ELIF r > 0.1 AND r <= 0.86 THEN a = .85 b = .04 c = -.04 d = .85 e = 0 f = 1.6 ELIF r > 0.86 AND r <= 0.93 THEN a = .2 b = -.26 c = .23 d = .22 e = 0 f = 1.6 ELIF r > 0.93 THEN a = -.15 b = .28 c = .26 d = .24 e = 0 f = .44 END IF newx = a * x + b * y + e newy = c * x + d * y + f x = newx y = newy emulate_move((int)(600 + 96 * x), (int)(32 + 96 * y)) emulate_draw((int)(600 + 96 * x), (int)(32 + 96 * y)) NEXT i INPUT wait$ end_screen()
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 2, 2014 22:58:48 GMT 1
That didn't work.
r = QRND(1) <--- gcc reports not a function
OR
r = QRND <--- compiles but does the same as the BaCon RANDOM().
|
|
|
Post by vovchik on Feb 2, 2014 23:14:02 GMT 1
Dear John, No. It does make a difference: SEED NOW DEF FN QRND = (float)(RANDOM(1000) / 1000.0) DECLARE r, s TYPE double FOR i = 1 TO 10 r = QRND s = RANDOM(1) PRINT "QRND: ", r, " RANDOM(1): ", s NEXT i
And the fern prog now works.  With kind regards, vovchik
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 2, 2014 23:19:09 GMT 1
I think the easiest way to solve this is for me to add the BBC (Brandy) RND function as well as the GETKEY / WAITKEY functions to the libbbc.so library. We still need to think about mouse events but that's for another day.
I tried the following and it still doesn't work. Can you post your fern.bac code?
' Fern
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS m SDL bbc PROTO init_screen, emulate_mode, emulate_origin, emulate_off, emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen, SDL_WM_SetCaption DECLARE a,b,c,d,e,f,x,y,r,newx, newy TYPE FLOATING
init_screen() SDL_WM_SetCaption("BaCon BBC Fern", 0) emulate_mode(31) emulate_origin(200, 100) emulate_off() emulate_gcol(0, 10) x=0 y=0 FOR i = 1 TO 80000 SEED NOW DEF FN QRND = (float)(RANDOM(1000) / 1000.0) r = QRND IF r <= 0.1 THEN a = 0 b = 0 c = 0 d = 0.16 e = 0 f = 0 ENDIF IF r > 0.1 AND r <= 0.86 THEN a = .85 b = .04 c = -.04 d = .85 e = 0 f = 1.6 ENDIF IF r > 0.86 AND r <= 0.93 THEN a = .2 b = -.26 c = .23 d = .22 e = 0 f = 1.6 ENDIF IF r> 0.93 THEN a = -.15 b = .28 c = .26 d = .24 e = 0 f = .44 ENDIF newx = a * x + b * y + e newy = c * x + d * y + f x = newx y = newy emulate_move(600 + 96 * x, 32 + 96 * y) emulate_draw(600 + 96 * x, 32 + 96 * y) NEXT i INPUT wait$ end_screen()
|
|
|
Post by vovchik on Feb 2, 2014 23:39:37 GMT 1
It should be the same as above....
' Fern
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS m SDL bbc PROTO init_screen, emulate_mode, emulate_origin, emulate_off, emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen, SDL_WM_SetCaption DECLARE i TYPE long DECLARE a,b,c,d,e,f,x,y,r,newx, newy TYPE float SEED NOW DEF FN QRND = (float)(RANDOM(1000) / 1000.0)
init_screen() SDL_WM_SetCaption("BaCon BBC Fern", 0) emulate_mode(31) emulate_origin(200, 100) emulate_off() emulate_gcol(0, 10) x = 0 y = 0 FOR i = 1 TO 80000 r = QRND IF r <= 0.1 THEN a = 0 b = 0 c = 0 d = 0.16 e = 0 f = 0 ELIF r > 0.1 AND r <= 0.86 THEN a = .85 b = .04 c = -.04 d = .85 e = 0 f = 1.6 ELIF r > 0.86 AND r <= 0.93 THEN a = .2 b = -.26 c = .23 d = .22 e = 0 f = 1.6 ELIF r > 0.93 THEN a = -.15 b = .28 c = .26 d = .24 e = 0 f = .44 END IF newx = a * x + b * y + e newy = c * x + d * y + f x = newx y = newy emulate_move((int)(600 + 96 * x), (int)(32 + 96 * y)) emulate_draw((int)(600 + 96 * x), (int)(32 + 96 * y)) NEXT i INPUT wait$ end_screen()
But I am on 32-bit Mint....
With kind regards, vovchik
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 2, 2014 23:48:39 GMT 1
Thank You! Your version works. (needed the vovchik touch) 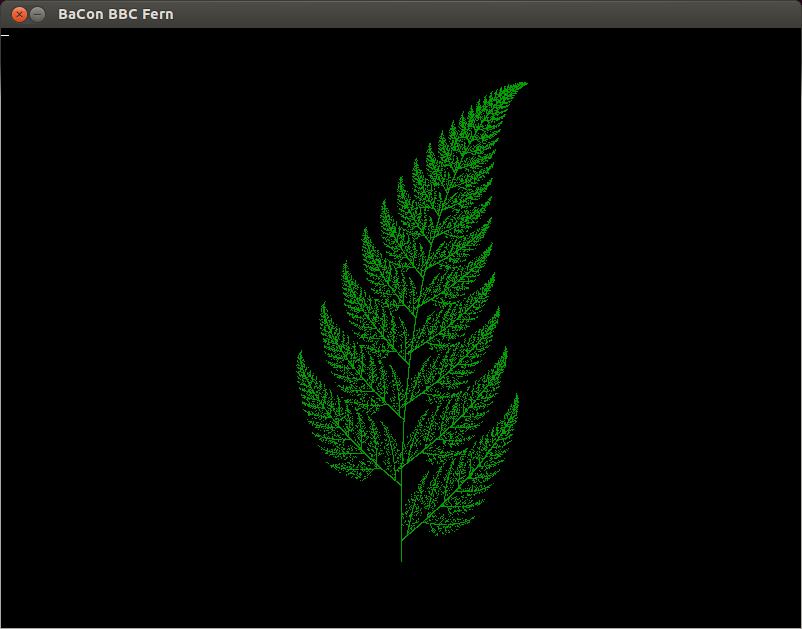
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 3, 2014 3:31:49 GMT 1
This is the polygon benchmark (1000 iterations) which doesn't seem to be working right. I've attached the original Brandy example as well. 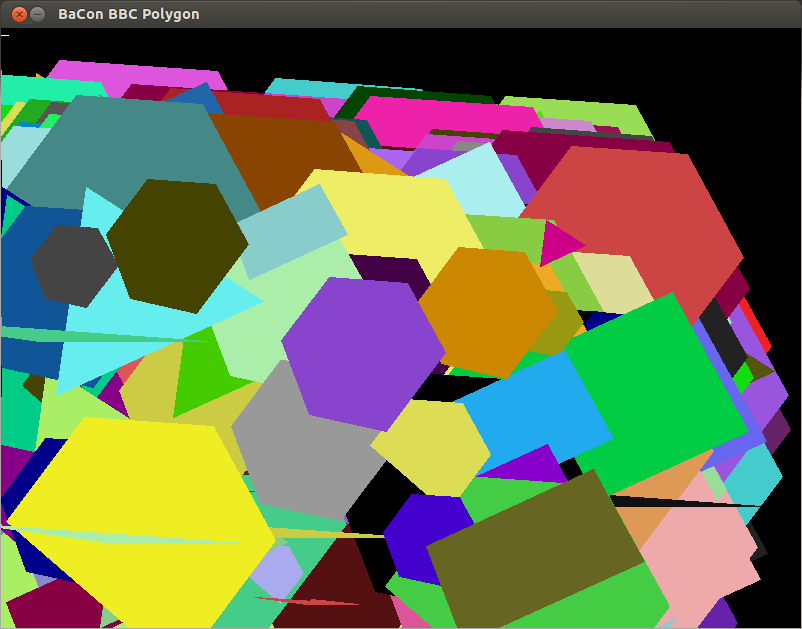 ' Polygon
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS m SDL bbc PROTO init_screen, emulate_mode, emulate_origin, emulate_off, emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen PROTO SDL_WM_SetCaption, emulate_newmode, emulate_vdu, emulate_plot, emulate_vdustr
DECLARE t1,t2,t3,width,height,i TYPE int DECLARE xorigin,yorigin,radius,c,d,l,t,side,sides,radius,angle GLOBAL x TYPE int ARRAY 10 GLOBAL y TYPE int ARRAY 10 SEED NOW
init_screen() SDL_WM_SetCaption("BaCon BBC Polygon", 0) t1 = SDL_GetTicks() emulate_newmode(800, 600, 256, -1) emulate_vdu(26) Width = 800 Height = 600 FOR i = 1 TO 1000 REM xorigin = BBC_RND(640 * 20) REM yorigin = BBC_RND(512 * 2) xorigin = RANDOM(1250) yorigin = RANDOM(840) radius = RANDOM(300) + 50 emulate_origin(xorigin, yorigin) sides = RANDOM(8) + 2 emulate_move(radius, 0) emulate_move(10, 10) c = RANDOM(64) - 1 t = (RANDOM(4) -1) << 6 emulate_gcol(0, c, t) FOR side = 1 TO sides angle = (side - 1) * 2 * PI / sides x[side] = radius * COS(angle) y[side] = radius * SIN(angle) emulate_move(0, 0) emulate_plot(85, x[side], y[side]) NEXT side emulate_move(0, 0) emulate_plot(85, radius, 0) REPEAT d = RANDOM(64) - 1 UNTIL (d AND 63)<>(c AND 6) emulate_gcol(0,d, t) FOR SIDE = 1 TO sides FOR l = side TO sides emulate_move(x[side], y[side]) emulate_draw(x[l], y[l]) NEXT l NEXT side NEXT i t2 = SDL_GetTicks() t3 = (t2-t1)/1000 emulate_off() REM emulate_vdustr("Time: " & STR$(t3) & " seconds.") INPUT wait$ end_screen()
This is what it's suppose to look like. polygon.bbv (1.11 KB)
|
|
|
Post by vovchik on Feb 3, 2014 10:50:11 GMT 1
Dear John, This seems to work.... The main problem was, I think, trivial (side/SIDE) in the last FOR/NEXT loop.  ' Polygon
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS SDL bbc PROTO init_screen, emulate_mode, emulate_origin, emulate_off, emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen PROTO SDL_WM_SetCaption, emulate_newmode, emulate_vdu, emulate_plot DECLARE angle, radius TYPE double DECLARE xorigin, yorigin, i, l TYPE int DECLARE c, t, d, side, sides TYPE int DECLARE x[10] TYPE double DECLARE y[10] TYPE double SEED NOW init_screen() SDL_WM_SetCaption("BaCon BBC Polygon", 0) emulate_newmode(800, 600, 256, -1) emulate_vdu(26) FOR i = 1 TO 1000 xorigin = RANDOM(1250) yorigin = RANDOM(840) radius = RANDOM(300) + 50 emulate_origin((int)xorigin, (int)yorigin) sides = RANDOM(8) + 2 emulate_move((int)radius, 0) emulate_move(10, 10) c = RANDOM(64) + 1 t = (RANDOM(4) - 1) << 6 emulate_gcol(0, (int)c, (int)t) FOR side = 1 TO sides angle = (double)(((side - 1) * 2) * (PI / sides)) x[side] = (double)(radius * COS(angle)) y[side] = (double)(radius * SIN(angle)) emulate_move(0, 0) emulate_plot(85, (int)x[side], (int)y[side]) NEXT side emulate_move(0, 0) emulate_plot(85, (int)radius, 0) REPEAT d = ABS(RANDOM(64) - 1) UNTIL (d & 63) <> (c & 6) emulate_gcol(0, (int)d, (int)t) FOR side = 1 TO sides FOR l = side TO sides emulate_move((int)x[side], (int)y[side]) emulate_draw((int)x[l], (int)y[l]) NEXT l NEXT side NEXT i INPUT wait$ end_screen()
With kind regards, vovchik
|
|
Deleted
Deleted Member
Posts: 0
|
Post by Deleted on Feb 3, 2014 16:19:45 GMT 1
Thanks for your efforts getting these examples working. The casting additions were a big surprise. I may still try to add a bbc_rnd function to the library. The BaCon RND isn't as random as I would like to see. (1 second seeding may be the issue) I'm seeing artifacts which indicates overflow errors. I'm wondering if there is anything Peter can do to prevent having to use C casting methods in the BASIC.? 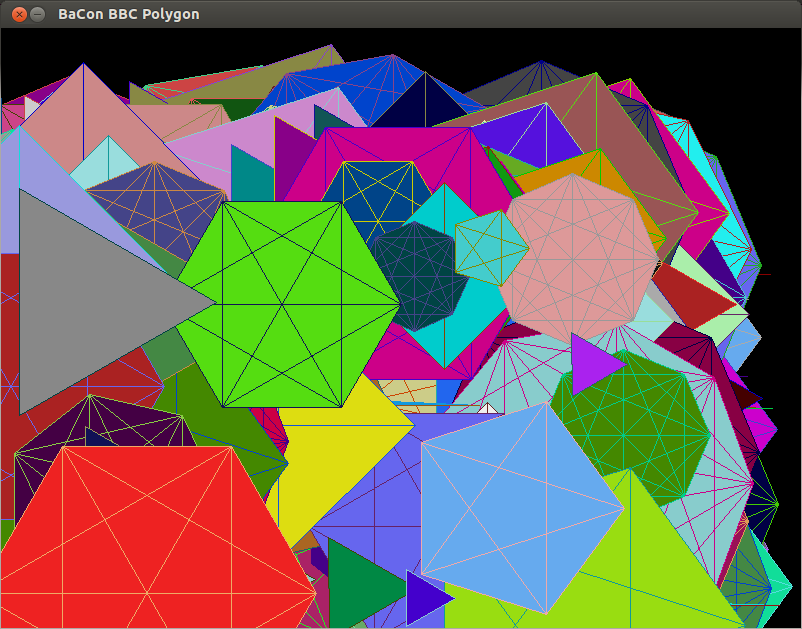
|
|
|
Post by bigbass on Feb 3, 2014 16:30:43 GMT 1
Thanks John and vovchik for the work However getting the Bacon syntax to work correctly should be studied using any of the working examples this one in particular has several places where it isn't easy to port correctly if you diff out the differences it will become clearer I am usually busy documenting things and I uploaded vovchik's example to the wiki and will upload more because also the forum eats the formatting and it is harder to follow the flow of the code without having to format it again Sdlpolygon.bacthanks again this is very useful and I learned a lot just reading the code  Joe
|
|
|
Post by vovchik on Feb 3, 2014 19:44:30 GMT 1
Dear all, I don't know whether this is standard practice with SDL, but I looked at one of Peter's SDL examples and cobbled together this method for closing the window: ' Polygon demo using BBC basic (brandy) lib with SDL calls
' *********************** ' COMPILER DIRECTIVES ' ***********************
PRAGMA INCLUDE SDL.h PRAGMA OPTIONS -I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT -DHAVE_OPENGL PRAGMA LDFLAGS SDL bbc
' *********************** ' END COMPILER DIRECTIVES ' ***********************
' *********************** ' EXTERNAL FUNCTIONS ' ***********************
PROTO init_screen, emulate_mode, emulate_origin, emulate_off PROTO emulate_gcol, emulate_off, emulate_draw, emulate_move, end_screen PROTO emulate_newmode, emulate_vdu, emulate_plot PROTO SDL_WM_SetCaption, SDL_PollEvent
' *********************** ' END EXTERNAL FUNCTIONS ' ***********************
' *********************** ' INITIALIZATION ' ***********************
' create some BBC aliases ALIAS "init_screen" TO "BBC_INIT" ALIAS "emulate_mode" TO "BBC_MODE" ALIAS "emulate_move" TO "BBC_MOVE" ALIAS "emulate_newmode" TO "BBC_NEWMODE" ALIAS "emulate_origin" TO "BBC_ORIGIN" ALIAS "emulate_gcol" TO "BBC_COLOR" ALIAS "emulate_origin" TO "BBC_ORIGIN" ALIAS "emulate_off" TO "BBC_ON" ALIAS "emulate_off" TO "BBC_OFF" ALIAS "emulate_draw" TO "BBC_DRAW" ALIAS "emulate_plot" TO "BBC_PLOT" ALIAS "end_screen" TO "BBC_END" ALIAS "emulate_vdu" TO "BBC_VDU" ALIAS "SDL_WM_SetCaption" TO "BBC_WINDOW_TITLE" ALIAS "SDL_PollEvent" TO "BBC_GETKEY" ' define var types DECLARE angle, radius TYPE double DECLARE xorigin, yorigin, i, l TYPE int DECLARE c, t, d, side, sides TYPE int DECLARE x[10] TYPE double DECLARE y[10] TYPE double ' initialize random number generator SEED NOW
' *********************** ' INITIALIZATION ' ***********************
' *********************** ' SUBS & FUNCTIONS ' ***********************
' ------------------ SUB BBC_WINDOW(int w, int h, int mode, int colours, STRING title$) ' ------------------ ' create main SDL display window BBC_INIT() BBC_NEWMODE(w, h, colours, -1) BBC_WINDOW_TITLE(title$, 0) BBC_VDU(mode) END SUB
' ------------------ SUB DRAW() ' ------------------ ' draw polygons FOR i = 1 TO 1000 xorigin = RANDOM(1250) yorigin = RANDOM(840) radius = RANDOM(300) + 50 BBC_ORIGIN((int)xorigin, (int)yorigin) sides = RANDOM(8) + 2 BBC_MOVE((int)radius, 0) BBC_MOVE(10, 10) c = RANDOM(64) + 1 t = (RANDOM(4) - 1) << 6 BBC_COLOR(0, (int)c, (int)t) FOR side = 1 TO sides angle = (double)(((side - 1) * 2) * (PI / sides)) x[side] = (double)(radius * COS(angle)) y[side] = (double)(radius * SIN(angle)) BBC_MOVE(0, 0) BBC_PLOT(85, (int)x[side], (int)y[side]) NEXT side BBC_MOVE(0, 0) BBC_PLOT(85, (int)radius, 0) REPEAT d = ABS(RANDOM(64) - 1) UNTIL (d & 63) <> (c & 6) BBC_COLOR(0, (int)d, (int)t) FOR side = 1 TO sides FOR l = side TO sides BBC_MOVE((int)x[side], (int)y[side]) BBC_DRAW((int)x[l], (int)y[l]) NEXT l NEXT side NEXT i END SUB
' ------------------ SUB MK_GUI() ' ------------------ ' create SDL window BBC_WINDOW(800, 600, 26, 256, "BBC-BACON Polygons (Hit <RETURN> to exit)") ' draw polygons DRAW() ' wait for user input before closing END SUB
' ------------------ SUB KEY_WAIT() ' ------------------ LOCAL event TYPE SDL_Event WHILE TRUE DO WHILE BBC_GETKEY(&event) DO SELECT event.type CASE SDL_KEYUP ' If return is pressed, quit ' see http://www.libsdl.org/release/SDL-1.2.15/docs/html/sdlkey.html IF event.key.keysym.sym = SDLK_RETURN THEN BBC_OFF() BBC_END() END 0 END IF CASE SDL_QUIT END 0 END SELECT WEND WEND END SUB
' *********************** ' END SUBS & FUNCTIONS ' ***********************
' *********************** ' MAIN ' ***********************
' draw polygons MK_GUI() ' wait for <RETURN> to close window KEY_WAIT()
' *********************** ' END MAIN ' ***********************
With kind regards, vovchik PS. See also this bit: www.aaroncox.net/tutorials/2dtutorials/sdlkeyboard.html
|
|